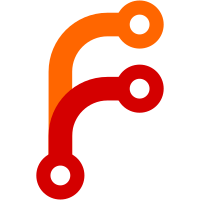
* First version of Vs. Seeker * Update movement.inc98f7e9978d (r1306721924)
* Update field_effect_scripts.s https://github.com/rh-hideout/pokeemerald-expansion/pull/3256/files#r1306722004 * Update field_effect_scripts.s https://github.com/rh-hideout/pokeemerald-expansion/pull/3256/files#r1306722024 * Update item_use.h https://github.com/rh-hideout/pokeemerald-expansion/pull/3256/files#r1306722401 * Update movement_action_func_tables.h https://github.com/rh-hideout/pokeemerald-expansion/pull/3256/files#r1306722828 * Update event_object_movement.c https://github.com/rh-hideout/pokeemerald-expansion/pull/3256/files#r1306722887 * Update overworld.c https://github.com/rh-hideout/pokeemerald-expansion/pull/3256/files#r1306723396 * Update vs_seeker.h https://github.com/rh-hideout/pokeemerald-expansion/pull/3256/files#r1306724158 * Update vs_seeker.c Addressed some cleanup comments from SBird * Update UpdateRandomTrainerRematches Fixed typo in ClearAllTrainerRematchStates Fixed types in GetRematchableTrainerLocalId * Updated UseVsSeekerEffect_2 * Updated UseVsSeekerEffect_3 * Updated UseVsSeekerEffect_4 * Fixed bug that allowed Vs Seeker to be used indoors in correct places Moved VsSeeker function declarations into header * Refactored FieldUseFunc_VsSeeker * Added curly braces to else case in FieldUseFunc_VsSeeker * renamed data[x] in Task_ResetObjectsRematchWantedState * Refactored Task_ResetObjectsRematchWantedState * Refactored VsSeekerResetObjectMovementAfterChargeComplete * Refactored ResetMovementOfRematchableTrainers * Refactored GatherNearbyTrainerInfo * Refactored Task_VsSeeker_3 * CanUseVsSeeker * Refactored GetVsSeekerResponseInArea * GetCurVsSeekerResponse refactored * Cleaned up GetTrainerFlagFromScript * Gave sensible names to Task_VsSeeker * Fixed two bugs where player would not have the right gfx state after using VsSeeker on a Bike or Underwater * Renamed UseVsSeeker Functions * Added I_VS_SEEKER_CHARGING to make Vs. Seeker broken until flag is assigned Removed extra VsSeeker animation code * Addressed PR feedback * Fixed issue with building non-modern * Refactored GetRunningBehaviorFromGraphicsId and renamed to GetResponseMovementTypeFromTrainerGraphicsId * Addresses Lunos's PR feedback: https://github.com/rh-hideout/pokeemerald-expansion/pull/3256\#pullrequestreview-1623547850 Removed the check to see if a map was not indoors to improve readability Made IsValidLocationForVsSeeker into a static function * Added changes in response to Jasper's feedback https://github.com/rh-hideout/pokeemerald-expansion/pull/3256\#pullrequestreview-1725276522 * Updated with Edu's discord feedback https://discord.com/channels/419213663107416084/1135040810082123907/1176872015085453392 * Removed ifdef tags around the repo unless neededb5dc744ced
77 lines
2.9 KiB
C
77 lines
2.9 KiB
C
#ifndef GUARD_BATTLE_SETUP_H
|
|
#define GUARD_BATTLE_SETUP_H
|
|
|
|
#include "gym_leader_rematch.h"
|
|
|
|
#define REMATCHES_COUNT 5
|
|
|
|
struct RematchTrainer
|
|
{
|
|
u16 trainerIds[REMATCHES_COUNT];
|
|
u16 mapGroup;
|
|
u16 mapNum;
|
|
};
|
|
|
|
extern const struct RematchTrainer gRematchTable[REMATCH_TABLE_ENTRIES];
|
|
|
|
extern u16 gTrainerBattleOpponent_A;
|
|
extern u16 gTrainerBattleOpponent_B;
|
|
extern u16 gPartnerTrainerId;
|
|
|
|
void BattleSetup_StartWildBattle(void);
|
|
void BattleSetup_StartDoubleWildBattle(void);
|
|
void BattleSetup_StartBattlePikeWildBattle(void);
|
|
void BattleSetup_StartRoamerBattle(void);
|
|
void StartWallyTutorialBattle(void);
|
|
void BattleSetup_StartScriptedWildBattle(void);
|
|
void BattleSetup_StartScriptedDoubleWildBattle(void);
|
|
void BattleSetup_StartLatiBattle(void);
|
|
void BattleSetup_StartLegendaryBattle(void);
|
|
void StartGroudonKyogreBattle(void);
|
|
void StartRegiBattle(void);
|
|
u8 BattleSetup_GetTerrainId(void);
|
|
u8 GetWildBattleTransition(void);
|
|
u8 GetTrainerBattleTransition(void);
|
|
u8 GetSpecialBattleTransition(s32 id);
|
|
void ChooseStarter(void);
|
|
void ResetTrainerOpponentIds(void);
|
|
void SetMapVarsToTrainer(void);
|
|
const u8 *BattleSetup_ConfigureTrainerBattle(const u8 *data);
|
|
void ConfigureAndSetUpOneTrainerBattle(u8 trainerObjEventId, const u8 *trainerScript);
|
|
void ConfigureTwoTrainersBattle(u8 trainerObjEventId, const u8 *trainerScript);
|
|
void SetUpTwoTrainersBattle(void);
|
|
bool32 GetTrainerFlagFromScriptPointer(const u8 *data);
|
|
void SetTrainerFacingDirection(void);
|
|
u8 GetTrainerBattleMode(void);
|
|
bool8 GetTrainerFlag(void);
|
|
bool8 HasTrainerBeenFought(u16 trainerId);
|
|
void SetTrainerFlag(u16 trainerId);
|
|
void ClearTrainerFlag(u16 trainerId);
|
|
void BattleSetup_StartTrainerBattle(void);
|
|
void BattleSetup_StartRematchBattle(void);
|
|
void ShowTrainerIntroSpeech(void);
|
|
const u8 *BattleSetup_GetScriptAddrAfterBattle(void);
|
|
const u8 *BattleSetup_GetTrainerPostBattleScript(void);
|
|
void ShowTrainerCantBattleSpeech(void);
|
|
void PlayTrainerEncounterMusic(void);
|
|
const u8 *GetTrainerALoseText(void);
|
|
const u8 *GetTrainerBLoseText(void);
|
|
const u8 *GetTrainerWonSpeech(void);
|
|
void UpdateRematchIfDefeated(s32 rematchTableId);
|
|
void IncrementRematchStepCounter(void);
|
|
void TryUpdateRandomTrainerRematches(u16 mapGroup, u16 mapNum);
|
|
bool32 DoesSomeoneWantRematchIn(u16 mapGroup, u16 mapNum);
|
|
bool32 IsRematchTrainerIn(u16 mapGroup, u16 mapNum);
|
|
u16 GetLastBeatenRematchTrainerId(u16 trainerId);
|
|
bool8 ShouldTryRematchBattle(void);
|
|
bool8 IsTrainerReadyForRematch(void);
|
|
void ShouldTryGetTrainerScript(void);
|
|
u16 CountBattledRematchTeams(u16 trainerId);
|
|
|
|
void DoStandardWildBattle_Debug(void);
|
|
void BattleSetup_StartTrainerBattle_Debug(void);
|
|
s32 TrainerIdToRematchTableId(const struct RematchTrainer *table, u16 trainerId);
|
|
s32 FirstBattleTrainerIdToRematchTableId(const struct RematchTrainer *table, u16 trainerId);
|
|
u16 GetRematchTrainerIdFromTable(const struct RematchTrainer *table, u16 firstBattleTrainerId);
|
|
|
|
#endif // GUARD_BATTLE_SETUP_H
|