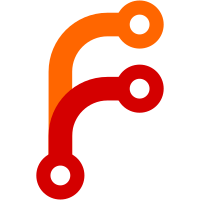
* Made gBattleMoves handle the InGame name and description of battle moves No more multiple arrays in separate, individual files. Note: -Keep an eye on Task_LearnedMove. * Reintroduced move names Misc: -Fixed Trick-or-Treat and Light of Ruin's expanded names. -Introduced a new field for Z-Move names, and a constant for their name length. -Added a few TODOs to GetBattleMoveName. -Updated GetMaxMoveName and GetZMoveName. There's no reason not to let GetBattleMoveName handle everything on its own. * Updated GetBattleMoveName to handle Z-Move Names Misc: -Removed pointless TODO about MOVE_NAME_LENGTH. -The compiler doesn't allow to have a move name with a value higher than MOVE_NAME_LENGTH, therefore it's pointless to worry about it. * Fixed a couple of expanded move names * Removed zMoveName variable of struct BattleMove and extended the name variable's size * Ditched no longer used MOVE_NAME_LENGTH constant * Corrected the names of the max moves I should have done this after updating the size of the name variable of the struct BattleMove, but I didn't think about it at all until Cancer Fairy indirectly gave me the idea. * Fixed U-turn's name * Brought back MOVE_NAME_LENGTH I think it doesn't make sense to have a Z_MOVE_NAME_LENGTH because the length in question is used for all battle moves, not just the Z-Moves. * Introduced a union for Move/Z-Move names in the struct BattleMove * Fixed the union for gBattleMoves move names Also updated GetBattleMoveName to properly handle Max Move names. Also also renamed the "zMoveName" variable to "bigMoveName" which better reflects its purpose. Z-Move names weren't the only thing it covered, since it also handles Max Move names. * Removed deprecated GetZMoveName and GetMaxMoveName * Reintroduced mention to gMoveNames in sGFRomHeader * Fixed move names and ported move descriptions * Fused the struct ContestMove into the struct BattleMove * Removed no longer used Z_MOVE_NAME_LENGTH constant * Renamed the struct BattleMove's bigMoveName variable and introduced macros to prettify move names * Reintroduced the contest parameters for Pokémon moves * Renamed gBattleMoves to gMovesInfo This is consistent with gSpeciesInfo, the array that contains most of the species data. * Renamed the BattleMove struct to MovesInfo This is consistent with the struct SpeciesInfo, which contains the variables used by the gSpeciesInfo array. * Removed empty lines separating battle params from contest params in gMovesInfo * Renamed MovesInfo to MoveInfo * Added Cancer Fairy's HANDLE_EXPANDED_MOVE_NAME macro Used to handle moves with expanded names in a more comfortable manner. Also fixed Trick-or-Treat's expanded name. * Renamed GetBattleMoveName to GetMoveName * Added a comment pointing out that the shared move descriptions are shared move descriptions * Re-aligned one of the escape characters of CHECK_MOVE_FLAG * Renamed the battle_moves.h file to moves_info.h instead for consistency's sake * Applied Eduardo's adjustments * Using compound string for regular move names as well, saving 1180 bytes and making their use consistent * Move description formatting * Updated Pursuit test after merge * Renamed the BATTLE_CATEGORY constants to DAMAGE_CATEGORY --------- Co-authored-by: Nephrite <thechurchofcage@gmail.com> Co-authored-by: Bassoonian <iasperbassoonian@gmail.com> Co-authored-by: Eduardo Quezada D'Ottone <eduardo602002@gmail.com>
98 lines
3.8 KiB
C
98 lines
3.8 KiB
C
#include "global.h"
|
|
#include "test/battle.h"
|
|
|
|
ASSUMPTIONS
|
|
{
|
|
ASSUME(gMovesInfo[MOVE_HOWL].effect == EFFECT_ATTACK_UP_USER_ALLY);
|
|
}
|
|
|
|
SINGLE_BATTLE_TEST("Howl raises user's Attack", s16 damage)
|
|
{
|
|
bool32 raiseAttack;
|
|
PARAMETRIZE { raiseAttack = FALSE; }
|
|
PARAMETRIZE { raiseAttack = TRUE; }
|
|
GIVEN {
|
|
ASSUME(gMovesInfo[MOVE_TACKLE].category == DAMAGE_CATEGORY_PHYSICAL);
|
|
PLAYER(SPECIES_WOBBUFFET);
|
|
OPPONENT(SPECIES_WOBBUFFET);
|
|
} WHEN {
|
|
if (raiseAttack) TURN { MOVE(player, MOVE_HOWL); }
|
|
TURN { MOVE(player, MOVE_TACKLE); }
|
|
} SCENE {
|
|
if (raiseAttack) {
|
|
ANIMATION(ANIM_TYPE_MOVE, MOVE_HOWL, player);
|
|
ANIMATION(ANIM_TYPE_GENERAL, B_ANIM_STATS_CHANGE, player);
|
|
MESSAGE("Wobbuffet's Attack rose!");
|
|
}
|
|
ANIMATION(ANIM_TYPE_MOVE, MOVE_TACKLE, player);
|
|
HP_BAR(opponent, captureDamage: &results[i].damage);
|
|
} FINALLY {
|
|
EXPECT_MUL_EQ(results[0].damage, Q_4_12(1.5), results[1].damage);
|
|
}
|
|
}
|
|
|
|
DOUBLE_BATTLE_TEST("Howl raises user's and partner's Attack", s16 damageLeft, s16 damageRight)
|
|
{
|
|
bool32 raiseAttack;
|
|
PARAMETRIZE { raiseAttack = FALSE; }
|
|
PARAMETRIZE { raiseAttack = TRUE; }
|
|
GIVEN {
|
|
ASSUME(gMovesInfo[MOVE_TACKLE].category == DAMAGE_CATEGORY_PHYSICAL);
|
|
PLAYER(SPECIES_WOBBUFFET) { Speed(15); }
|
|
PLAYER(SPECIES_WYNAUT) { Speed(10); }
|
|
OPPONENT(SPECIES_WOBBUFFET) { Speed(13); }
|
|
OPPONENT(SPECIES_WYNAUT) { Speed(12); }
|
|
} WHEN {
|
|
if (raiseAttack) TURN { MOVE(playerLeft, MOVE_HOWL); }
|
|
TURN { MOVE(playerLeft, MOVE_TACKLE, target: opponentLeft); }
|
|
TURN { MOVE(playerRight, MOVE_TACKLE, target: opponentRight); }
|
|
} SCENE {
|
|
if (raiseAttack) {
|
|
ANIMATION(ANIM_TYPE_MOVE, MOVE_HOWL, playerLeft);
|
|
ANIMATION(ANIM_TYPE_GENERAL, B_ANIM_STATS_CHANGE, playerLeft);
|
|
MESSAGE("Wobbuffet's Attack rose!");
|
|
ANIMATION(ANIM_TYPE_GENERAL, B_ANIM_STATS_CHANGE, playerRight);
|
|
MESSAGE("Wynaut's Attack rose!");
|
|
}
|
|
ANIMATION(ANIM_TYPE_MOVE, MOVE_TACKLE, playerLeft);
|
|
HP_BAR(opponentLeft, captureDamage: &results[i].damageLeft);
|
|
ANIMATION(ANIM_TYPE_MOVE, MOVE_TACKLE, playerRight);
|
|
HP_BAR(opponentRight, captureDamage: &results[i].damageRight);
|
|
} FINALLY {
|
|
EXPECT_MUL_EQ(results[0].damageLeft, Q_4_12(1.5), results[1].damageLeft);
|
|
EXPECT_MUL_EQ(results[0].damageRight, Q_4_12(1.5), results[1].damageRight);
|
|
}
|
|
}
|
|
|
|
DOUBLE_BATTLE_TEST("Howl does not work on partner if it has Soundproof")
|
|
{
|
|
s16 damage[2];
|
|
|
|
GIVEN {
|
|
ASSUME(gMovesInfo[MOVE_TACKLE].category == DAMAGE_CATEGORY_PHYSICAL);
|
|
PLAYER(SPECIES_WOBBUFFET) { Speed(15); }
|
|
PLAYER(SPECIES_VOLTORB) { Speed(10); Ability(ABILITY_SOUNDPROOF); }
|
|
OPPONENT(SPECIES_WOBBUFFET) { Speed(5); }
|
|
OPPONENT(SPECIES_WYNAUT) { Speed(1); }
|
|
} WHEN {
|
|
TURN { MOVE(playerRight, MOVE_TACKLE, target: opponentLeft); }
|
|
TURN { MOVE(playerLeft, MOVE_HOWL); MOVE(playerRight, MOVE_TACKLE, target: opponentLeft); }
|
|
} SCENE {
|
|
ANIMATION(ANIM_TYPE_MOVE, MOVE_TACKLE, playerRight);
|
|
HP_BAR(opponentLeft, captureDamage: &damage[0]);
|
|
|
|
ANIMATION(ANIM_TYPE_MOVE, MOVE_HOWL, playerLeft);
|
|
ANIMATION(ANIM_TYPE_GENERAL, B_ANIM_STATS_CHANGE, playerLeft);
|
|
MESSAGE("Wobbuffet's Attack rose!");
|
|
NONE_OF {
|
|
ANIMATION(ANIM_TYPE_GENERAL, B_ANIM_STATS_CHANGE, playerRight);
|
|
MESSAGE("Wynaut's Attack rose!");
|
|
}
|
|
ABILITY_POPUP(playerRight, ABILITY_SOUNDPROOF);
|
|
MESSAGE("Voltorb's Soundproof blocks Howl!");
|
|
ANIMATION(ANIM_TYPE_MOVE, MOVE_TACKLE, playerRight);
|
|
HP_BAR(opponentLeft, captureDamage: &damage[1]);
|
|
} THEN {
|
|
EXPECT_EQ(damage[0], damage[1]);
|
|
}
|
|
}
|