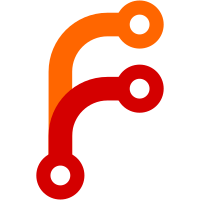
* settwoturnstring command * Unified two-turn attacks and Meteor Beam To do: Solar Beam * Solar Beam Also fixed various function, removed EFFECT_GUST (who knows why that exists?) * Updated Solar Beam + tests * Redid two turn move + animations logic Removed pointless various function; to do: remove Skull Bash, fix AI test * Removed now-pointless flag * Removed Skull Bash And temporarily commented out failing AI tests * Removed Sky Uppercut effect Not sure when or why this was ever necessary * Removed BattleScript_EffectSemiInvulnerable Now uses BattleScript_EffectTwoTurnsAttack. Kept the effect; used the argument field to determine which STATUS3 such moves should apply but added a function to jump over weather checks in BattleScript_EffectTwoTurnsAttack if the current move is semi-invulnerable (since the instant-fire weather check and STATUS3 use the same field) * Applied review changes * Replaced VARIOUS with callnative Tried to fix test but couldn't :/ wtf is going on * Fixed one AI test Cant fix the other... * Added KNOWN_FAILING to failing AI tests Separated them out into their own test * Optimised script, overhauled charge turn string setting Condensed multiple confusing macros into one, jumpifweathercheckchargeeffects. Script now tweaked and trimmed, string ids for charge turns now added to argument along with status3 (thanks to compression macro) and instant-fire-weather for semi-invulnerable and two-turn moves respectively. Also introduced a savedStringId in gBattleScripting to make string selection work. * Unified two turn move tests + minor corrections * Added semi-invulnerable move tests Set the Razor Wind test to known failing - something to do with its animation? --------- Co-authored-by: Alex <93446519+AlexOn1ine@users.noreply.github.com>
250 lines
8.8 KiB
C
250 lines
8.8 KiB
C
#include "global.h"
|
|
#include "test/battle.h"
|
|
|
|
ASSUMPTIONS
|
|
{
|
|
ASSUME(gMovesInfo[MOVE_FLY].effect == EFFECT_SEMI_INVULNERABLE);
|
|
ASSUME(UNCOMPRESS_BITS(HIHALF(gMovesInfo[MOVE_FLY].argument)) == STATUS3_ON_AIR);
|
|
ASSUME(gMovesInfo[MOVE_DIG].effect == EFFECT_SEMI_INVULNERABLE);
|
|
ASSUME(UNCOMPRESS_BITS(HIHALF(gMovesInfo[MOVE_DIG].argument)) == STATUS3_UNDERGROUND);
|
|
ASSUME(gMovesInfo[MOVE_BOUNCE].effect == EFFECT_SEMI_INVULNERABLE);
|
|
ASSUME(UNCOMPRESS_BITS(HIHALF(gMovesInfo[MOVE_BOUNCE].argument)) == STATUS3_ON_AIR);
|
|
ASSUME(gMovesInfo[MOVE_DIVE].effect == EFFECT_SEMI_INVULNERABLE);
|
|
ASSUME(UNCOMPRESS_BITS(HIHALF(gMovesInfo[MOVE_DIVE].argument)) == STATUS3_UNDERWATER);
|
|
ASSUME(gMovesInfo[MOVE_PHANTOM_FORCE].effect == EFFECT_SEMI_INVULNERABLE);
|
|
ASSUME(UNCOMPRESS_BITS(HIHALF(gMovesInfo[MOVE_PHANTOM_FORCE].argument)) == STATUS3_PHANTOM_FORCE);
|
|
ASSUME(gMovesInfo[MOVE_SHADOW_FORCE].effect == EFFECT_SEMI_INVULNERABLE);
|
|
ASSUME(UNCOMPRESS_BITS(HIHALF(gMovesInfo[MOVE_SHADOW_FORCE].argument)) == STATUS3_PHANTOM_FORCE);
|
|
}
|
|
|
|
SINGLE_BATTLE_TEST("Semi-invulnerable moves make the user semi-invulnerable turn 1, then strike turn 2")
|
|
{
|
|
u16 move;
|
|
|
|
PARAMETRIZE { move = MOVE_FLY; }
|
|
PARAMETRIZE { move = MOVE_DIG; }
|
|
PARAMETRIZE { move = MOVE_BOUNCE; }
|
|
PARAMETRIZE { move = MOVE_DIVE; }
|
|
PARAMETRIZE { move = MOVE_PHANTOM_FORCE; }
|
|
PARAMETRIZE { move = MOVE_SHADOW_FORCE; }
|
|
|
|
GIVEN {
|
|
PLAYER(SPECIES_WOBBUFFET);
|
|
OPPONENT(SPECIES_WOBBUFFET);
|
|
} WHEN {
|
|
TURN { MOVE(player, move); MOVE(opponent, MOVE_AERIAL_ACE); }
|
|
TURN { SKIP_TURN(player); }
|
|
} SCENE {
|
|
// Charging turn
|
|
if (B_UPDATED_MOVE_DATA >= GEN_5)
|
|
{
|
|
switch (move)
|
|
{
|
|
case MOVE_FLY:
|
|
NOT MESSAGE("Wobbuffet flew up high!");
|
|
MESSAGE("Wobbuffet used Fly!");
|
|
break;
|
|
case MOVE_DIG:
|
|
NOT MESSAGE("Wobbuffet dug a hole!");
|
|
MESSAGE("Wobbuffet used Dig!");
|
|
break;
|
|
case MOVE_BOUNCE:
|
|
NOT MESSAGE("Wobbuffet sprang up!");
|
|
MESSAGE("Wobbuffet used Bounce!");
|
|
break;
|
|
case MOVE_DIVE:
|
|
NOT MESSAGE("Wobbuffet hid underwater!");
|
|
MESSAGE("Wobbuffet used Dive!");
|
|
break;
|
|
case MOVE_PHANTOM_FORCE:
|
|
NOT MESSAGE("Wobbuffet vanished instantly!");
|
|
MESSAGE("Wobbuffet used PhantomForce!");
|
|
break;
|
|
case MOVE_SHADOW_FORCE:
|
|
NOT MESSAGE("Wobbuffet vanished instantly!");
|
|
MESSAGE("Wobbuffet used Shadow Force!");
|
|
break;
|
|
}
|
|
} else {
|
|
ANIMATION(ANIM_TYPE_MOVE, move, player);
|
|
}
|
|
if (B_UPDATED_MOVE_DATA < GEN_5)
|
|
{
|
|
switch (move)
|
|
{
|
|
case MOVE_FLY:
|
|
MESSAGE("Wobbuffet flew up high!");
|
|
break;
|
|
case MOVE_DIG:
|
|
MESSAGE("Wobbuffet dug a hole!");
|
|
break;
|
|
case MOVE_BOUNCE:
|
|
MESSAGE("Wobbuffet sprang up!");
|
|
break;
|
|
case MOVE_DIVE:
|
|
MESSAGE("Wobbuffet hid underwater!");
|
|
break;
|
|
case MOVE_PHANTOM_FORCE:
|
|
case MOVE_SHADOW_FORCE:
|
|
MESSAGE("Wobbuffet vanished instantly!");
|
|
break;
|
|
}
|
|
}
|
|
else
|
|
ANIMATION(ANIM_TYPE_MOVE, move, player);
|
|
|
|
// Aerial Ace cannot miss unless the target is semi-invulnerable
|
|
MESSAGE("Foe Wobbuffet used Aerial Ace!");
|
|
MESSAGE("Foe Wobbuffet's attack missed!");
|
|
// Attack turn
|
|
switch (move)
|
|
{
|
|
case MOVE_FLY:
|
|
MESSAGE("Wobbuffet used Fly!");
|
|
break;
|
|
case MOVE_DIG:
|
|
MESSAGE("Wobbuffet used Dig!");
|
|
break;
|
|
case MOVE_BOUNCE:
|
|
MESSAGE("Wobbuffet used Bounce!");
|
|
break;
|
|
case MOVE_DIVE:
|
|
MESSAGE("Wobbuffet used Dive!");
|
|
break;
|
|
case MOVE_PHANTOM_FORCE:
|
|
MESSAGE("Wobbuffet used PhantomForce!");
|
|
break;
|
|
case MOVE_SHADOW_FORCE:
|
|
MESSAGE("Wobbuffet used Shadow Force!");
|
|
break;
|
|
}
|
|
ANIMATION(ANIM_TYPE_MOVE, move, player);
|
|
HP_BAR(opponent);
|
|
}
|
|
}
|
|
|
|
SINGLE_BATTLE_TEST("Semi-invulnerable moves don't need to charge with Power Herb")
|
|
{
|
|
u16 move;
|
|
|
|
PARAMETRIZE { move = MOVE_FLY; }
|
|
PARAMETRIZE { move = MOVE_DIG; }
|
|
PARAMETRIZE { move = MOVE_BOUNCE; }
|
|
PARAMETRIZE { move = MOVE_DIVE; }
|
|
PARAMETRIZE { move = MOVE_PHANTOM_FORCE; }
|
|
PARAMETRIZE { move = MOVE_SHADOW_FORCE; }
|
|
|
|
GIVEN {
|
|
PLAYER(SPECIES_WOBBUFFET) { Item(ITEM_POWER_HERB); }
|
|
OPPONENT(SPECIES_WOBBUFFET);
|
|
} WHEN {
|
|
TURN { MOVE(player, move); }
|
|
} SCENE {
|
|
// Charging turn
|
|
if (B_UPDATED_MOVE_DATA >= GEN_5)
|
|
{
|
|
switch (move)
|
|
{
|
|
case MOVE_FLY:
|
|
NOT MESSAGE("Wobbuffet flew up high!");
|
|
MESSAGE("Wobbuffet used Fly!");
|
|
break;
|
|
case MOVE_DIG:
|
|
NOT MESSAGE("Wobbuffet dug a hole!");
|
|
MESSAGE("Wobbuffet used Dig!");
|
|
break;
|
|
case MOVE_BOUNCE:
|
|
NOT MESSAGE("Wobbuffet sprang up!");
|
|
MESSAGE("Wobbuffet used Bounce!");
|
|
break;
|
|
case MOVE_DIVE:
|
|
NOT MESSAGE("Wobbuffet hid underwater!");
|
|
MESSAGE("Wobbuffet used Dive!");
|
|
break;
|
|
case MOVE_PHANTOM_FORCE:
|
|
NOT MESSAGE("Wobbuffet vanished instantly!");
|
|
MESSAGE("Wobbuffet used PhantomForce!");
|
|
break;
|
|
case MOVE_SHADOW_FORCE:
|
|
NOT MESSAGE("Wobbuffet vanished instantly!");
|
|
MESSAGE("Wobbuffet used Shadow Force!");
|
|
break;
|
|
}
|
|
} else {
|
|
ANIMATION(ANIM_TYPE_MOVE, move, player);
|
|
}
|
|
if (B_UPDATED_MOVE_DATA < GEN_5)
|
|
{
|
|
switch (move)
|
|
{
|
|
case MOVE_FLY:
|
|
MESSAGE("Wobbuffet flew up high!");
|
|
break;
|
|
case MOVE_DIG:
|
|
MESSAGE("Wobbuffet dug a hole!");
|
|
break;
|
|
case MOVE_BOUNCE:
|
|
MESSAGE("Wobbuffet sprang up!");
|
|
break;
|
|
case MOVE_DIVE:
|
|
MESSAGE("Wobbuffet hid underwater!");
|
|
break;
|
|
case MOVE_PHANTOM_FORCE:
|
|
case MOVE_SHADOW_FORCE:
|
|
MESSAGE("Wobbuffet vanished instantly!");
|
|
break;
|
|
}
|
|
}
|
|
else
|
|
ANIMATION(ANIM_TYPE_MOVE, move, player);
|
|
MESSAGE("Wobbuffet became fully charged due to its Power Herb!");
|
|
if (B_UPDATED_MOVE_DATA < GEN_5)
|
|
{
|
|
switch (move)
|
|
{
|
|
case MOVE_FLY:
|
|
MESSAGE("Wobbuffet used Fly!");
|
|
break;
|
|
case MOVE_DIG:
|
|
MESSAGE("Wobbuffet used Dig!");
|
|
break;
|
|
case MOVE_BOUNCE:
|
|
MESSAGE("Wobbuffet used Bounce!");
|
|
break;
|
|
case MOVE_DIVE:
|
|
MESSAGE("Wobbuffet used Dive!");
|
|
break;
|
|
case MOVE_PHANTOM_FORCE:
|
|
MESSAGE("Wobbuffet used PhantomForce!");
|
|
break;
|
|
case MOVE_SHADOW_FORCE:
|
|
MESSAGE("Wobbuffet used Shadow Force!");
|
|
break;
|
|
}
|
|
}
|
|
ANIMATION(ANIM_TYPE_MOVE, move, player);
|
|
HP_BAR(opponent);
|
|
}
|
|
}
|
|
|
|
// No way to apply this test with Shadow Force/Phantom Force
|
|
SINGLE_BATTLE_TEST("Semi-invulnerable moves apply a status that won't block certain moves")
|
|
{
|
|
u16 move, opMove;
|
|
|
|
PARAMETRIZE { move = MOVE_FLY; opMove = MOVE_SKY_UPPERCUT; }
|
|
PARAMETRIZE { move = MOVE_DIG; opMove = MOVE_EARTHQUAKE; }
|
|
PARAMETRIZE { move = MOVE_BOUNCE; opMove = MOVE_THUNDER; }
|
|
PARAMETRIZE { move = MOVE_DIVE; opMove = MOVE_SURF; }
|
|
|
|
GIVEN {
|
|
PLAYER(SPECIES_WOBBUFFET);
|
|
OPPONENT(SPECIES_WOBBUFFET);
|
|
} WHEN {
|
|
TURN { MOVE(player, move); MOVE(opponent, opMove); }
|
|
} SCENE {
|
|
ANIMATION(ANIM_TYPE_MOVE, move, player);
|
|
ANIMATION(ANIM_TYPE_MOVE, opMove, opponent);
|
|
HP_BAR(player);
|
|
}
|
|
}
|