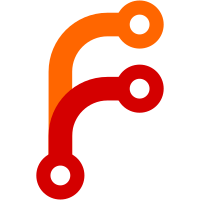
In contests, even FONT_NARROWER isn't sufficient to prevent clipping in all cases. e.g. Stomping Tantrum clips. We have decided to accept that cost to make the rest of the user experience better, but downstream projects that don't like that trade-off can either a) alter the contest UI, or b) set B_EXPANDED_MOVE_NAMES to FALSE.
118 lines
3.9 KiB
C
118 lines
3.9 KiB
C
#include "global.h"
|
|
#include "test/battle.h"
|
|
|
|
// From https://bulbapedia.bulbagarden.net/wiki/Damage#Example
|
|
|
|
SINGLE_BATTLE_TEST("Damage calculation matches Gen5+")
|
|
{
|
|
s16 dmg;
|
|
s16 expectedDamage;
|
|
PARAMETRIZE { expectedDamage = 196; }
|
|
PARAMETRIZE { expectedDamage = 192; }
|
|
PARAMETRIZE { expectedDamage = 192; }
|
|
PARAMETRIZE { expectedDamage = 192; }
|
|
PARAMETRIZE { expectedDamage = 184; }
|
|
PARAMETRIZE { expectedDamage = 184; }
|
|
PARAMETRIZE { expectedDamage = 184; }
|
|
PARAMETRIZE { expectedDamage = 180; }
|
|
PARAMETRIZE { expectedDamage = 180; }
|
|
PARAMETRIZE { expectedDamage = 180; }
|
|
PARAMETRIZE { expectedDamage = 172; }
|
|
PARAMETRIZE { expectedDamage = 172; }
|
|
PARAMETRIZE { expectedDamage = 172; }
|
|
PARAMETRIZE { expectedDamage = 168; }
|
|
PARAMETRIZE { expectedDamage = 168; }
|
|
PARAMETRIZE { expectedDamage = 168; }
|
|
GIVEN {
|
|
ASSUME(gMovesInfo[MOVE_ICE_FANG].category == DAMAGE_CATEGORY_PHYSICAL);
|
|
PLAYER(SPECIES_GLACEON) { Level(75); Attack(123); }
|
|
OPPONENT(SPECIES_GARCHOMP) { Defense(163); }
|
|
} WHEN {
|
|
TURN {
|
|
MOVE(player, MOVE_ICE_FANG, WITH_RNG(RNG_DAMAGE_MODIFIER, i));
|
|
}
|
|
}
|
|
SCENE {
|
|
MESSAGE("Glaceon used Ice Fang!");
|
|
HP_BAR(opponent, captureDamage: &dmg);
|
|
}
|
|
THEN {
|
|
EXPECT_EQ(expectedDamage, dmg);
|
|
}
|
|
}
|
|
|
|
SINGLE_BATTLE_TEST("Damage calculation matches Gen5+ (Muscle Band, crit)")
|
|
{
|
|
s16 dmg;
|
|
s16 expectedDamage;
|
|
PARAMETRIZE { expectedDamage = 324; }
|
|
PARAMETRIZE { expectedDamage = 316; }
|
|
PARAMETRIZE { expectedDamage = 312; }
|
|
PARAMETRIZE { expectedDamage = 312; }
|
|
PARAMETRIZE { expectedDamage = 304; }
|
|
PARAMETRIZE { expectedDamage = 304; }
|
|
PARAMETRIZE { expectedDamage = 300; }
|
|
PARAMETRIZE { expectedDamage = 300; }
|
|
PARAMETRIZE { expectedDamage = 292; }
|
|
PARAMETRIZE { expectedDamage = 292; }
|
|
PARAMETRIZE { expectedDamage = 288; }
|
|
PARAMETRIZE { expectedDamage = 288; }
|
|
PARAMETRIZE { expectedDamage = 280; }
|
|
PARAMETRIZE { expectedDamage = 276; }
|
|
PARAMETRIZE { expectedDamage = 276; }
|
|
PARAMETRIZE { expectedDamage = 268; }
|
|
GIVEN {
|
|
ASSUME(gMovesInfo[MOVE_ICE_FANG].category == DAMAGE_CATEGORY_PHYSICAL);
|
|
PLAYER(SPECIES_GLACEON) { Level(75); Attack(123); Item(ITEM_MUSCLE_BAND); }
|
|
OPPONENT(SPECIES_GARCHOMP) { Defense(163); }
|
|
} WHEN {
|
|
TURN {
|
|
MOVE(player, MOVE_ICE_FANG, WITH_RNG(RNG_DAMAGE_MODIFIER, i), criticalHit: TRUE);
|
|
}
|
|
}
|
|
SCENE {
|
|
MESSAGE("Glaceon used Ice Fang!");
|
|
HP_BAR(opponent, captureDamage: &dmg);
|
|
}
|
|
THEN {
|
|
EXPECT_EQ(expectedDamage, dmg);
|
|
}
|
|
}
|
|
|
|
SINGLE_BATTLE_TEST("Damage calculation matches Gen5+ (Marshadow vs Mawile)")
|
|
{
|
|
s16 dmg;
|
|
s16 expectedDamage;
|
|
PARAMETRIZE { expectedDamage = 145; }
|
|
PARAMETRIZE { expectedDamage = 144; }
|
|
PARAMETRIZE { expectedDamage = 142; }
|
|
PARAMETRIZE { expectedDamage = 141; }
|
|
PARAMETRIZE { expectedDamage = 139; }
|
|
PARAMETRIZE { expectedDamage = 138; }
|
|
PARAMETRIZE { expectedDamage = 136; }
|
|
PARAMETRIZE { expectedDamage = 135; }
|
|
PARAMETRIZE { expectedDamage = 133; }
|
|
PARAMETRIZE { expectedDamage = 132; }
|
|
PARAMETRIZE { expectedDamage = 130; }
|
|
PARAMETRIZE { expectedDamage = 129; }
|
|
PARAMETRIZE { expectedDamage = 127; }
|
|
PARAMETRIZE { expectedDamage = 126; }
|
|
PARAMETRIZE { expectedDamage = 124; }
|
|
PARAMETRIZE { expectedDamage = 123; }
|
|
GIVEN {
|
|
ASSUME(gMovesInfo[MOVE_SPECTRAL_THIEF].category == DAMAGE_CATEGORY_PHYSICAL);
|
|
PLAYER(SPECIES_MARSHADOW) { Level(100); Attack(286); }
|
|
OPPONENT(SPECIES_MAWILE) { Level(100); Defense(226); HP(241); }
|
|
} WHEN {
|
|
TURN {
|
|
MOVE(player, MOVE_SPECTRAL_THIEF, WITH_RNG(RNG_DAMAGE_MODIFIER, i), criticalHit: FALSE);
|
|
}
|
|
}
|
|
SCENE{
|
|
MESSAGE("Marshadow used Spectral Thief!");
|
|
HP_BAR(opponent, captureDamage: &dmg);
|
|
}
|
|
THEN{
|
|
EXPECT_EQ(expectedDamage, dmg);
|
|
}
|
|
}
|