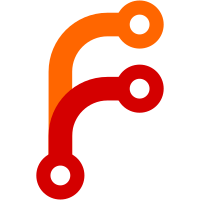
* SwitchAI makes much smarter mon choices
* Add HasHadOdds check to ShouldSwitch decision
* Remove early return
* Rework Baton Pass check as per discussion with Alex
* Forgot to adjust a comment
* Don't program before breakfast lol (if / else if fix)
* Switch AI_CalcDamage for AI_DATA->simulatedDmg in HasBadOdds
Thanks Alex! :D
* Typo in a hitToKO comparison
* Remove and replace AI_CalcPartyMonBestMoveDamage and IsAiPartyMonOHKOBy from https://github.com/rh-hideout/pokeemerald-expansion/pull/3146
See https://discord.com/channels/419213663107416084/1144447521960251472 for details
* Major refactor, new struct, switchin considers damage / healing from hazards / status / held item / weather
* Forgot Snow exists and heals Ice Body, haven't played Switch games lol
* (766a1a27a7
) Compatibility, use new struct field instead of function call
* Fixing oversight from previous upstream merge
* Improve TSpikes handling to make GetSwitchinHazardDamage more applicable
Small fixes:
- EFFECT_EXPLOSION typo (!= to ==)
- Order of if statements near bestResistEffective
- Spacing of terms in big HasBadOdds if statements
* Forgot to uncomment blocks disabled for debugging what turned out to be vanilla behaviour lol
* Remove another holdover from debugging, sorry :/
* Lastly, undoing my debug trainer
* Type matchup based on species type rather than current type
Suggested by BLourenco on Discord, the idea is that a mon that's had its type affected by a move like Soak will still have moves as though it was its regular typing, and so prioritizing the temporary typing wouldn't be ideal.
https://discord.com/channels/419213663107416084/1144447521960251472/1146644578141736970
* gActiveBattler upcoming merge fixes
* Egg changes part 1
* Egg changes part 2, just need to address EWRAM still
* Move SwitchinCandidate struct to AiLogicData
* Consider Steel type when checking TSpikes
* Comment about CanBePoisoned compatibility
* Changes for Egg's 2nd review
* Put period back in comment, whoops lol
* Latest upcoming merge fixes
* Missed a few u32 updates
* Combine GetBestMonIntegrate functions / flags, some modularization
* Fix merge error
* Make modern fixes
* Two tests done, two to go
* Accidentally pushed reference test, removing it
* Type matchup switching tests
* Tests for defensive vs offense switches
---------
Co-authored-by: DizzyEggg <jajkodizzy@wp.pl>
59 lines
2.9 KiB
C
59 lines
2.9 KiB
C
#ifndef GUARD_CONSTANTS_BATTLE_AI_H
|
|
#define GUARD_CONSTANTS_BATTLE_AI_H
|
|
|
|
// battlers
|
|
#define AI_TARGET 0
|
|
#define AI_USER 1
|
|
#define AI_TARGET_PARTNER 2
|
|
#define AI_USER_PARTNER 3
|
|
|
|
// get_type command
|
|
#define AI_TYPE1_TARGET 0
|
|
#define AI_TYPE1_USER 1
|
|
#define AI_TYPE2_TARGET 2
|
|
#define AI_TYPE2_USER 3
|
|
#define AI_TYPE_MOVE 4
|
|
|
|
// type effectiveness
|
|
#define AI_EFFECTIVENESS_x8 7
|
|
#define AI_EFFECTIVENESS_x4 6
|
|
#define AI_EFFECTIVENESS_x2 5
|
|
#define AI_EFFECTIVENESS_x1 4
|
|
#define AI_EFFECTIVENESS_x0_5 3
|
|
#define AI_EFFECTIVENESS_x0_25 2
|
|
#define AI_EFFECTIVENESS_x0_125 1
|
|
#define AI_EFFECTIVENESS_x0 0
|
|
|
|
// AI Flags. Most run specific functions to update score, new flags are used for internal logic in other scripts
|
|
#define AI_FLAG_CHECK_BAD_MOVE (1 << 0)
|
|
#define AI_FLAG_TRY_TO_FAINT (1 << 1)
|
|
#define AI_FLAG_CHECK_VIABILITY (1 << 2)
|
|
#define AI_FLAG_SETUP_FIRST_TURN (1 << 3)
|
|
#define AI_FLAG_RISKY (1 << 4)
|
|
#define AI_FLAG_PREFER_STRONGEST_MOVE (1 << 5)
|
|
#define AI_FLAG_PREFER_BATON_PASS (1 << 6)
|
|
#define AI_FLAG_DOUBLE_BATTLE (1 << 7) // removed, split between AI_FLAG_CHECK_BAD_MOVE & AI_FLAG_CHECK_GOOD_MOVE
|
|
#define AI_FLAG_HP_AWARE (1 << 8)
|
|
// New, Trainer Handicap Flags
|
|
#define AI_FLAG_NEGATE_UNAWARE (1 << 9) // AI is NOT aware of negating effects like wonder room, mold breaker, etc
|
|
#define AI_FLAG_WILL_SUICIDE (1 << 10) // AI will use explosion / self destruct / final gambit / etc
|
|
// New, Trainer Strategy Flags
|
|
#define AI_FLAG_HELP_PARTNER (1 << 11) // AI can try to help partner. If not set, will tend not to target partner
|
|
#define AI_FLAG_PREFER_STATUS_MOVES (1 << 12) // AI gets a score bonus for status moves. Should be combined with AI_FLAG_CHECK_BAD_MOVE to prevent using only status moves
|
|
#define AI_FLAG_STALL (1 << 13) // AI stalls battle and prefers secondary damage/trapping/etc. TODO not finished
|
|
#define AI_FLAG_SCREENER (1 << 14) // AI prefers screening effects like reflect, mist, etc. TODO unfinished
|
|
#define AI_FLAG_SMART_SWITCHING (1 << 15) // AI includes a lot more switching checks
|
|
#define AI_FLAG_ACE_POKEMON (1 << 16) // AI has an Ace Pokemon. The last Pokemon in the party will not be used until it's the last one remaining.
|
|
#define AI_FLAG_OMNISCIENT (1 << 17) // AI has full knowledge of player moves, abilities, hold items
|
|
#define AI_FLAG_SMART_MON_CHOICES (1 << 18) // AI will make smarter decisions when choosing which mon to send out mid-battle and after a KO, which are separate decisions. Pairs very well with AI_FLAG_SMART_SWITCHING.
|
|
|
|
#define AI_FLAG_COUNT 18
|
|
|
|
// 'other' ai logic flags
|
|
#define AI_FLAG_ROAMING (1 << 29)
|
|
#define AI_FLAG_SAFARI (1 << 30)
|
|
#define AI_FLAG_FIRST_BATTLE (1 << 31)
|
|
|
|
#define AI_SCORE_DEFAULT 100 // Default score for all AI moves.
|
|
|
|
#endif // GUARD_CONSTANTS_BATTLE_AI_H
|