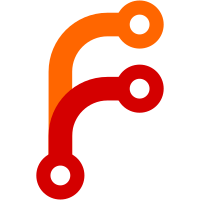
The title says most of it. For authentication, the token gotten from the POST method can be put as a 'Token' header. It's then validated before being used. Other changes caused by this update are: - Fixed a bug where the creation token was unusable due to lacking the user password hash - Changed the signing method to HMAC, requiring a string for encryption This is mentioned in the README.md
51 lines
1,000 B
Go
51 lines
1,000 B
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
_ "github.com/joho/godotenv/autoload"
|
|
"log"
|
|
"net/http"
|
|
"os"
|
|
)
|
|
|
|
type App struct {
|
|
UserHandler *UserHandler
|
|
}
|
|
|
|
// Define the serve function
|
|
func (h *App) ServeHTTP(res http.ResponseWriter, req *http.Request) {
|
|
var head string
|
|
head, req.URL.Path = ShiftPath(req.URL.Path)
|
|
switch head {
|
|
// Start the user handler should the requested user be found
|
|
case "user":
|
|
h.UserHandler.Handle(res, req)
|
|
// Return a `Not Found` if the user is not found
|
|
default:
|
|
http.Error(res, "Not Found", http.StatusNotFound)
|
|
}
|
|
}
|
|
|
|
// Run the server
|
|
func main() {
|
|
// Initialise the user handler
|
|
user_handler, err := NewUserHandler()
|
|
|
|
// Log any errors
|
|
if err != nil {
|
|
log.Fatalln(err)
|
|
}
|
|
|
|
a := &App{
|
|
UserHandler: user_handler,
|
|
}
|
|
|
|
port := os.Getenv("PORT")
|
|
if port == "" {
|
|
port = "7741"
|
|
}
|
|
// Log that the program has successfully started listening to the port
|
|
log.Println(fmt.Sprintf("Ambition backend listening to port %v", port))
|
|
http.ListenAndServe(":"+port, a)
|
|
}
|