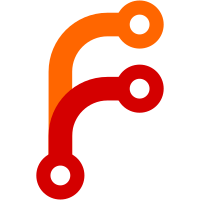
Former-commit-id: bfa963ac13e76b7e3cf2eb2e23651eb3189d3cbb Former-commit-id: 6602c3b0a22327d5ceb85063c9934b3490e1da01
34 lines
618 B
Rust
34 lines
618 B
Rust
#[derive(Debug, Primitive, PartialEq, Eq, Clone, Copy)]
|
|
#[repr(u8)]
|
|
pub enum Keys {
|
|
ButtonA = 0,
|
|
ButtonB = 1,
|
|
Select = 2,
|
|
Start = 3,
|
|
Right = 4,
|
|
Left = 5,
|
|
Up = 6,
|
|
Down = 7,
|
|
ButtonR = 8,
|
|
ButtonL = 9,
|
|
}
|
|
|
|
pub const NUM_KEYS: usize = 10;
|
|
pub const KEYINPUT_ALL_RELEASED: u16 = 0b1111111111;
|
|
|
|
#[derive(Debug, Primitive, PartialEq, Eq)]
|
|
#[repr(u8)]
|
|
pub enum KeyState {
|
|
Pressed = 0,
|
|
Released = 1,
|
|
}
|
|
|
|
impl Into<bool> for KeyState {
|
|
fn into(self) -> bool {
|
|
match self {
|
|
KeyState::Pressed => false,
|
|
KeyState::Released => true,
|
|
}
|
|
}
|
|
}
|